Do you want a clean code, in line with the best practices? The one with proper indentations and all other prescribed rules? Then keep on reading!
This article is for all of you who shudder upon seeing a strike-through function call, a class with a warning of the function, or a class being deprecated. I completely understand the obsessive-compulsive urge to clean up this kind of code even if it doesn’t really create any problems nor it will in the near future.
Of course, in order to see these messages, you need to use a proper editor and configure it correctly. Since it requires a whole new blog post, I won’t be focusing on it today.
Note: Bear in mind that all the screenshots in this article come from PhpStorm that is probably the most widely used tool out there.
Now, let’s focus on replacing the deprecated code.
The Registry Class Replacement
Most of the deprecated functions are easily replaceable, like the following:

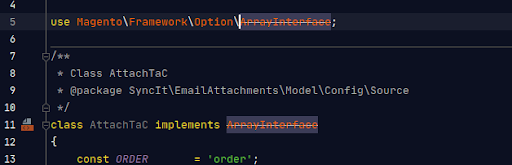
It’s enough to open the Reference classes. There, you’ll see the instructions for replacing the deprecated code. In most cases, the replacement code is backward compatible and doesn’t cause any issues (as with the examples below):
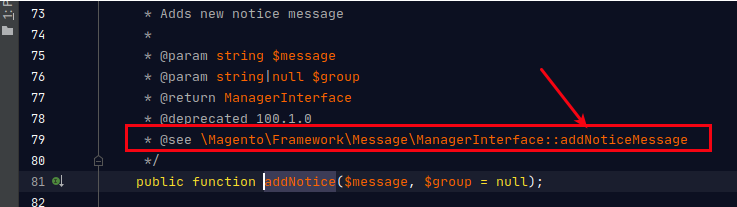
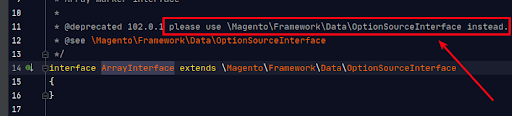
There are a few instances where the deprecated code is very frequent. However, there are still no clear instructions on how to replace it. One such, glaring, instance is the Registry class which is all over the place and has been deprecated for years:
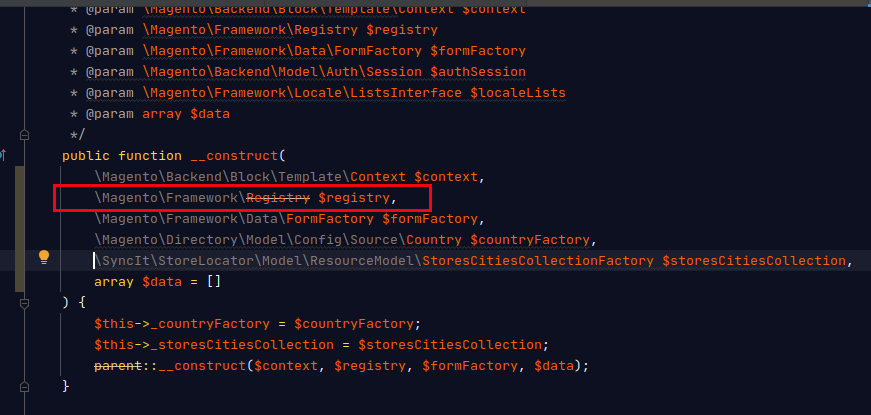
Reasons for Using the Registry Class
And there are two reasons you’ll need this class in your constructor.
In the first case, you’re extending a Magento or some custom module class that uses Registry in the constructor. So, you need to add your own (dependency-injected) classes in which case parent constructor arguments need to be re-declared. There’s nothing you can do here except change the way you’re injecting the classes you need.
Moreover, you’ll have to do it in the sense you don’t actually change them, but instead, use a paradigm labeled composition over inheritance.
Explaining how to do this is out of the scope of this article, but you can check out this great article written by one of the Magento masters if you’re interested in the subject.
The second reason for using a Registry class is for passing over global variables (those that will persist between two-page loads) between different classes/functions either for convenience or necessity (for example fetching current category or current product on the respective category and product page).
And what does Magento say about replacing Registry?
Let’s see.
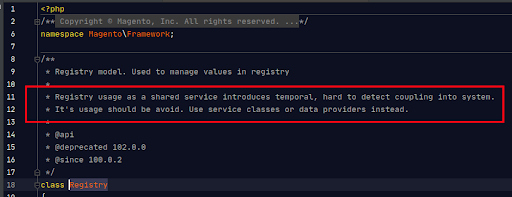
Not much, right? Just some general guidelines to use Service classes or data providers. But, the trouble is there’s no such thing as Service class or Data Provider class types, per se (DataProvider classes actually do exist but in a somewhat different context with UI Components). You have to create them yourself.
What exactly should a Service class be able to do?
For starters, it should be able to persist data. The natural place to do this is the user session and actually, Magento has even done a lot of heavy lifting here by providing us with just the class we can extend and use almost effortlessly instead of Registry.
So, to create your own ‘private’ Registry, you need to extend this class by creating your own module’s Session class:
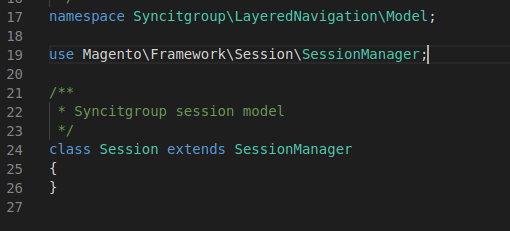
Then, you need to define a service class like this:
<?php
/**
* Syncitgroup Group
*
* This source file is subject to the Syncitgroup Software License, which is available at https://www.syncitgroup.com/.
* Do not edit or add to this file if you wish to upgrade to the newer versions in the future.
* If you wish to customize this module for your needs,
* please refer to http://www.magentocommerce.com for more information.
*
* @category Syncitgroup
* @package Syncitgroup_LayeredNavigation
* @author Martin Babic [email protected]
* @copyright 2020 (C) Syncitgroup (https://www.syncitgroup.com/)
* @license http://opensource.org/licenses/gpl-license.php GNU Public License
* @link https://www.syncitgroup.com/
*/
namespace Syncitgroup\LayeredNavigation\Service;
use Magento\Framework\Serialize\Serializer\Json;
use Psr\Log\LoggerInterface;
use Syncitgroup\LayeredNavigation\Model\Session;
class DataStorage
{
/**
* @var Session
*/
private $session;
/**
* @var Json
*/
private $jsonHelper;
/**
* @var LoggerInterface
*/
private $logger;
/**
* ControlValues constructor.
*
* @param Session $session
* @param Json $jsonHelper
* @param LoggerInterface $logger
*/
public function __construct(
Session $session,
Json $jsonHelper,
LoggerInterface $logger
) {
$this->session = $session;
$this->jsonHelper = $jsonHelper;
$this->logger = $logger;
}
/**
* Set arbitrary value
*
* @param string $name
* @param mixed $value
*/
public function setValue(string $name, $value)
{
$this->session->setData($name, $value);
}
/**
* Get value
*
* @param string $name
* @return mixed
*/
public function getValue(string $name)
{
return $this->session->getData($name);
}
/**
* Retrieve the value and immediately reset it (unregister it if you will)
*
* @param string $name
* @return mixed
*/
public function getValueAndReset(string $name)
{
return $this->session->getData($name, true);
}
/**
* Set arbitrary value in json format
*
* @param string $name
* @param mixed $value
*/
public function setJsonValue(string $name, $value)
{
$this->setValue($name, $this->jsonHelper->serialize($value));
}
/**
* Get value
*
* @param string $name
* @return mixed
*/
public function getJsonValue(string $name)
{
return $this->jsonHelper->unserialize($this->session->getData($name) ?: '[]');
}
}
Now, you can inject this service class DataStorage wherever you need it and use its getters and setters to share variables between different pieces of code.
So, instead of Register::register($varName, $val) you would call Service/DataStorage::setValue($varName, $val), etc.
Wrap Up
Feeling better now that you can get a clean code with the Registry class? Well, I’m glad I was able to help you out.
For more useful advice, keep on following our blog and feel free to contact us at [email protected]
Meanwhile, you can explore our favorite blog category.