The Role of Mobile Devices in eCommerce
Over the years, mobile devices have become more of a necessity rather than an accessory. Not long ago, mobile devices were used only for communication. Nowadays, mobile devices are everywhere. We use them for a variety of purposes such as transportation, paying taxes, navigation, and the like. Mobile app solutions have been growing quickly at an exponential rate. Of course, one of the fastest-growing mobile app usages is in eCommerce.
Due to the ease that mobile devices bring to the process of online shopping, eCommerce is becoming more and more popular by the second. In 2019, the number of American digital buyers was estimated at 215 million.
The Benefits of Mobile eCommerce
Mobile users are using their devices more and more to help them purchase various products they find appealing. Those products can range from clothing, furniture, electronics, to even groceries. These users now have the ability to browse and discover various products on-the-go. They can also easily access product reviews by previous purchasers on their mobile devices before making purchases. This information can have a large influence on potential customers whether they want to spend their money or not.
Mobile eCommerce apps allow their users to purchase online and get the wanted products at their doorstep. The benefit of using mobile eCommerce apps is their usage regardless of time and space. You can use them on the bus, in the shopping mall, while waiting at the airport, etc. On the contrary, desktop applications limit their users to use them from only one place, be it at home or at work. Many companies can benefit from selling their products even when their stores’ working time is up. This can help increase sales by removing certain purchasing limitations.
Importance of eCommerce Mobile App Marketing
Over the years, a mobile app has become an important strategy for many brands since it is able to boost brand recognition and drive revenue if utilized optimally. However, in order to take full advantage of having an eCommerce app, brands should be strategic in executing their app marketing campaigns. Whether it is to acquire new loyal users who will make purchases, retarget purchasers in old or existing apps to promote the new app, or re-engage lapsed users – programmatic advertising can help.
Magento 2 REST API
Magento exposes different REST endpoints depending on the type of user making the requests. It is crucial to request and include a security token in order to gain access to the Customer and Admin REST API endpoints. Magento REST API calls play an important role in mCommerce application development for Magento 2 websites. Every necessary API call must be handled with some of the mobile networking libraries I will be describing later in this text.
Synchronous Endpoints
Each of the following links leads to a list of REST endpoints specific to a user type:
- Admin REST API endpoints – Available using an admin security token.
- Customer REST API endpoints – Available using a customer security token
- Guest REST API endpoints – Available for anonymous users
Asynchronous Endpoints
You can run POST and PUT endpoints asynchronously while the async.operations.all message queue consumer is active. Asynchronous Web Endpoints provide information about asynchronous routes, payloads, and responses.
- Admin REST API endpoints – Available using an admin security token.
- Customer REST API endpoints – Available using a customer security token
- Guest REST API endpoints – Available for anonymous users
IOS and Android Libraries for Networking
Since mCommerce application needs to be in constant conjunction with REST API of the eCommerce website, in our case Magento 2, HTTP networking libraries will be needed to do so. In the following paragraph, we will describe mobile networking libraries for handling REST API calls.
Alamofire
Alamofire is an HTTP networking library written in Swift for devices that use iOS.
Requirements:
- iOS 10.0+ / macOS 10.12+ / tvOS 10.0+ / watchOS 3.0+
- Xcode 10.2+
- Swift 5+
Installation
CocoaPods
CocoaPods is a dependency manager for Cocoa projects. For usage and installation instructions, visit their website. To integrate Alamofire into your Xcode project using CocoaPods, specify it in your Podfile:
pod 'Alamofire', '~> 5.0.0-rc.3'
Carthage
Carthage is a decentralized dependency manager. It builds your dependencies and provides you with binary frameworks. In order to integrate Alamofire into your Xcode project using Carthage, you need to specify it in your Cartfile:
github "Alamofire/Alamofire" "5.0.0-rc.3"
Swift Package Manager
The Swift Package Manager is a tool for automating the distribution of Swift code and is integrated into the swift compiler. It is in early development, but Alamofire does support its use on supported platforms.
Once you have your Swift package set up, adding Alamofire as a dependency is as easy as adding it to the dependencies value of your Package.swift.
dependencies: [
.package(url: "https://github.com/Alamofire/Alamofire.git", from: "5.0.0-rc.3")
]
Manually
If you prefer not to use any of the aforementioned dependency managers, you can integrate Alamofire into your project manually.
Embedded Framework
- Open up Terminal, cd into your top-level project directory, and run the following command if your project is not initialized as a git repository:
$ git init
- Add Alamofire as a git submodule by running the following command:
$ git submodule add https://github.com/Alamofire/Alamofire.git
- Firstly, open the new Alamofire folder. Then, drag the Alamofire.xcodeproj into the Project Navigator of your application’s Xcode project. It should appear nested underneath your application’s blue project icon. Whether it is above or below all the other Xcode groups does not matter.
- Secondly, select the Alamofire.xcodeproj in the Project Navigator and verify the deployment target matches that of your application target.
- Thirdly, select your application project in the Project Navigator (blue project icon) to navigate to the target configuration window and the application target under the Targets heading in the sidebar.
- Then, in the tab bar at the top of that window, open the General panel.
- Click on the + button under the Embedded Binaries section.
- As a result, you will see two different Alamofire.xcodeproj folders each with two different versions of the Alamofire.framework nested inside a Products folder. It doesn’t matter which Products folder you choose from, but it does matter whether you choose the top or bottom Alamofire.framework.
- Finally, select the top Alamofire.framework for iOS and the bottom one for macOS. Also, you can verify which one you selected by inspecting the build log for your project. The build target for Alamofire will be listed as either Alamofire iOS, Alamofire macOS, Alamofire tvOS, or Alamofire watchOS.
- And that’s it!
The Alamofire.framework is automatically added as a target dependency, linked framework and embedded framework in a copy files build phase which is all you need to build on the simulator and a device.
Here is an example from our mCommerce application:
static func getSingleCategory(categoryId: String, completion: @escaping (_ category: Category) -> Void) {
AF.request(Urls.shared.singleCategoryUrl(categoryId: categoryId), method: .get, headers: AFHelper.getHeader(token: TokenManager.getAdminToken())).response { response in
self.printResponse(response: response)
if let data = response.data {
let decoder = JSONDecoder()
do {
let categories = try decoder.decode(Category.self, from: data)
//print(options)
completion(categories)
} catch {
print(error.localizedDescription)
}
}
}
}
Retrofit 2
Retrofit is a REST Client for Java and Android. It makes it easy to retrieve and upload JSON or XML via a REST-based web service. In Retrofit, you can configure which converter is used for the data serialization. Typically for JSON, you use GSon, but you can add custom converters to process XML or other protocols. Retrofit uses the OkHttp library for HTTP requests.
How to use Retrofit
In order to work with Retrofit, it is necessary to define a model class that is serialized to JSON. Then, you have to define Interface that defines the possible HTTP operations. And finally, you have to define Retrofit. Builder class – Instance which uses the interface and the Builder API to allow defining the URL endpoint for the HTTP operations.
Every method of an interface represents one possible API call. It must have an HTTP annotation (e.g., GET, POST, etc.) to specify the request type and the relative URL. The return value wraps the response in a Call object with the type of the expected result.
@GET
Single<OrdersResponse> getRecentOrdersRX(@Url String url);
You can call this method from another class, as shown in the following example:
public void getRecentOrders(CompositeDisposable disposable, MutableLiveData<OrdersResponse> ordersMutableLiveData, MutableLiveData<Boolean> loading, String customerId) {
disposable.add(RetrofitHelper.getInstance().getApi(true).getRecentOrdersRX(Urls.shared.myRecentOrders(customerId))
.subscribeOn(Schedulers.newThread())
.observeOn(AndroidSchedulers.mainThread())
.subscribeWith(new DisposableSingleObserver<OrdersResponse>() {
@Override
public void onSuccess(OrdersResponse ordersResponse) {
ordersMutableLiveData.setValue(ordersResponse);
loading.setValue(false);
}
@Override
public void onError(Throwable e) {
loading.setValue(false);
e.printStackTrace();
}
}
));
}
Authentication with OkHttp Interceptors
If you have more calls that require authentication, you can use an interceptor for this. An interceptor modifies each request prior to the performance and alters the request header. The advantage is that there is no need for adding the @Header(“Authorization”) to each API method definition.
The code below checks whether httpClient has or hasn’t got our interceptor.
static <S> S createService(boolean admin) {
AuthenticationInterceptor interceptor = new AuthenticationInterceptor(admin);
if (!httpClient.interceptors().contains(interceptor)) {
httpClient.addInterceptor(interceptor);
}
if (!httpClient.interceptors().contains(logging)) {
httpClient.addInterceptor(logging);
}
builder.client(httpClient.build());
retrofit = builder.build();
return retrofit.create((Class<S>) RetrofitApi.class);
}
In order to define your own Interceptor, you, too, can create a custom class AuthenticationInterceptor and override its intercept function as we have done in our app.
import java.io.IOException;
import okhttp3.Interceptor;
import okhttp3.Request;
import okhttp3.Response;
class AuthenticationInterceptor implements Interceptor {
boolean admin;
AuthenticationInterceptor(boolean admin) {
this.admin = admin;
}
@Override
public Response intercept(Chain chain) throws IOException {
Further in this code, a bearer token is added to the header of the HTTP request. Thus, authentication is done.
Example of an eCommerce Application
Here is one of many examples of eCommerce applications for purchasing different kinds of products. Usually, the navigation bar is necessary for guiding users through the application. Also, they should be able to see all the categories and products of the selected category. These can be seen inside of some fragment, in case of Android application, or in View Controller, in case of iOS application. Of course, cart review and payment information are also mandatory for any kind of eCommerce applications.
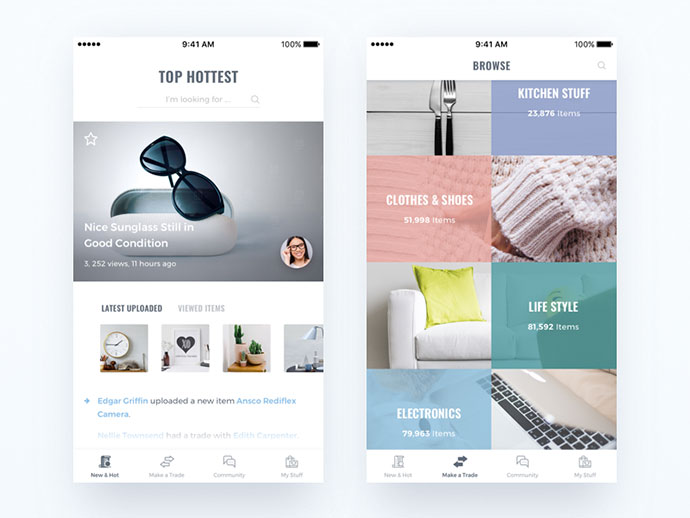
Naturally, safety is a must. So, any kind of payment should be as secure as possible.
Conclusion
In our mCommerce application, the mobile app communicates with Magento 2 REST API. Any inside action results in calling some network calls by networking libraries. Moreover, the implementation of this kind of application also requires solving a lot of security issues such as safe buying and safe ordering.
Our company is currently in the process of developing an amazing mCommerce application. So, stay tuned for the upcoming app news and the release date for both iOS and Android versions.
Sources